Creating CRUD Functionality for Cosmos SDK Custom Message Types
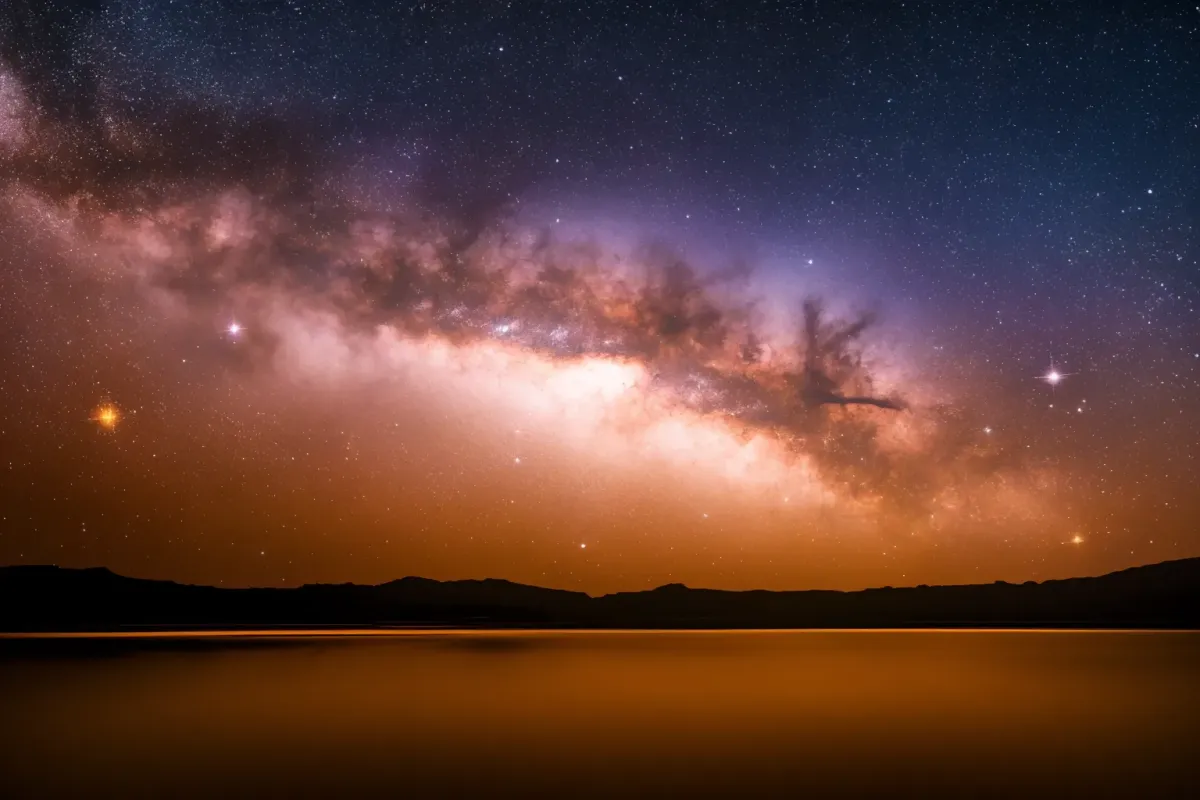
In our previous tutorialLink, we created a custom message type, but it had a few limitations, namely:
- It does not update a data store.
- It cannot be deleted from the data store.
- There is no straightforward way to look it up from the binary other than by using the transaction hash.
The third point is particularly problematic, as it limits the ability to query the data you need, leaving you to sift through heaps of transactions to find your data.
The solution is to scaffold a list
instead of a message
.
For this tutorial, we will continue using the same JediRegistry.
First, let's quit our blockchain using the q
command.
Note: If you did not complete the last tutorial, you can scaffold the chain with this command: ignite chain scaffold JediRegistry --address-prefix jedi
.
Below, we will scaffold a list. This command is exactly the same as in our last tutorial, but we have changed message
to list
and renamed registerJedi
to regJedi
:
ignite scaffold list regJedi name homePlanet forcePower:uint
After running this command, a long list of files will be created.
Next, launch your chain:
ignite chain serve
Once the chain is running, open another terminal window and check the available transactions:
JediRegistryd tx jediregistry --help
You should see the following transaction commands available:
create-reg-jedi
- Creates a regJedi entry.delete-reg-jedi
- Deletes a regJedi entry.register-jedi
- Sends a registerJedi transaction (previously created in the last tutorial).update-reg-jedi
- Updates a regJedi entry.
Creating and Managing Jedi in the Registry
Let's start by creating a Jedi in the registry:
JediRegistryd tx jediregistry create-reg-jedi "Anakin Skywalker" "Tatooine" 1000 --from yoda
Now, let's create one more Jedi:
JediRegistryd tx jediregistry create-reg-jedi "Luke Skywalker" "Tatooine" 777 --from yoda
You can look up a Jedi by the order they were created, starting with zero. Note that queries do not need to be signed, so there is no need for the --from yoda
flag:
JediRegistryd query jediregistry get-reg-jedi 0
You can also list all of the Jedi in the registry:
JediRegistryd query jediregistry list-reg-jedi
You should see that "Luke Skywalker" has an ID of 1.
Deleting a Jedi from the Registry
Unfortunately, Anakin has turned to the Dark Side and needs to be removed from the registry. Let's see what we need to do to accomplish this by checking the parameters required with the --help
flag:
JediRegistryd tx jediregistry delete-reg-jedi --help
You will see that you need the ID for Anakin, which is 0, and you will need to sign this transaction since it changes the state. It takes a Jedi Master like Yoda to submit and sign the transaction:
JediRegistryd tx jediregistry delete-reg-jedi 0 --from yoda
Now, check if the registry was updated:
JediRegistryd query jediregistry list-reg-jedi
Adding and Querying a New Jedi
Now, what happens if we check to see if we can get Anakin by ID after his registry entry was deleted?
JediRegistryd query jediregistry get-reg-jedi 0
Next, let's test what the ID will be if we add another Jedi. Will it be 0 or 2? There's only one way to find out:
JediRegistryd tx jediregistry create-reg-jedi "Rey Skywalker" "Tatooine" 450 --from yoda
JediRegistryd query jediregistry list-reg-jedi
The answer is 2
.
Now you can see the power of the SDK. Only Yoda was able to add Jedi. But what if Alice doesn't think Rey belongs and tries to delete her:
JediRegistryd tx jediregistry delete-reg-jedi 2 --from alice
We can see if she was successful:
JediRegistryd query jediregistry list-reg-jedi
Congratulations, you have successfully learned and implemented CRUD functionality for a Cosmos SDK chain!